This page will guide you to integrate and verify Contlo SDK in your Android application.
Prerequisites
To start the SDK integration, you must first create an API key and install the Mobile Push app from the Contlo App market. Go to your Contlo Dashboard and follow the steps below:
Create an API key
- Go to your profile section at the top right corner and click Settings.
- Go to Accounts > Store Settings from the sidebar on the Settings homepage.
- Go to the API keys tab on the Store Settings page and click Create key on the Private API keys section.
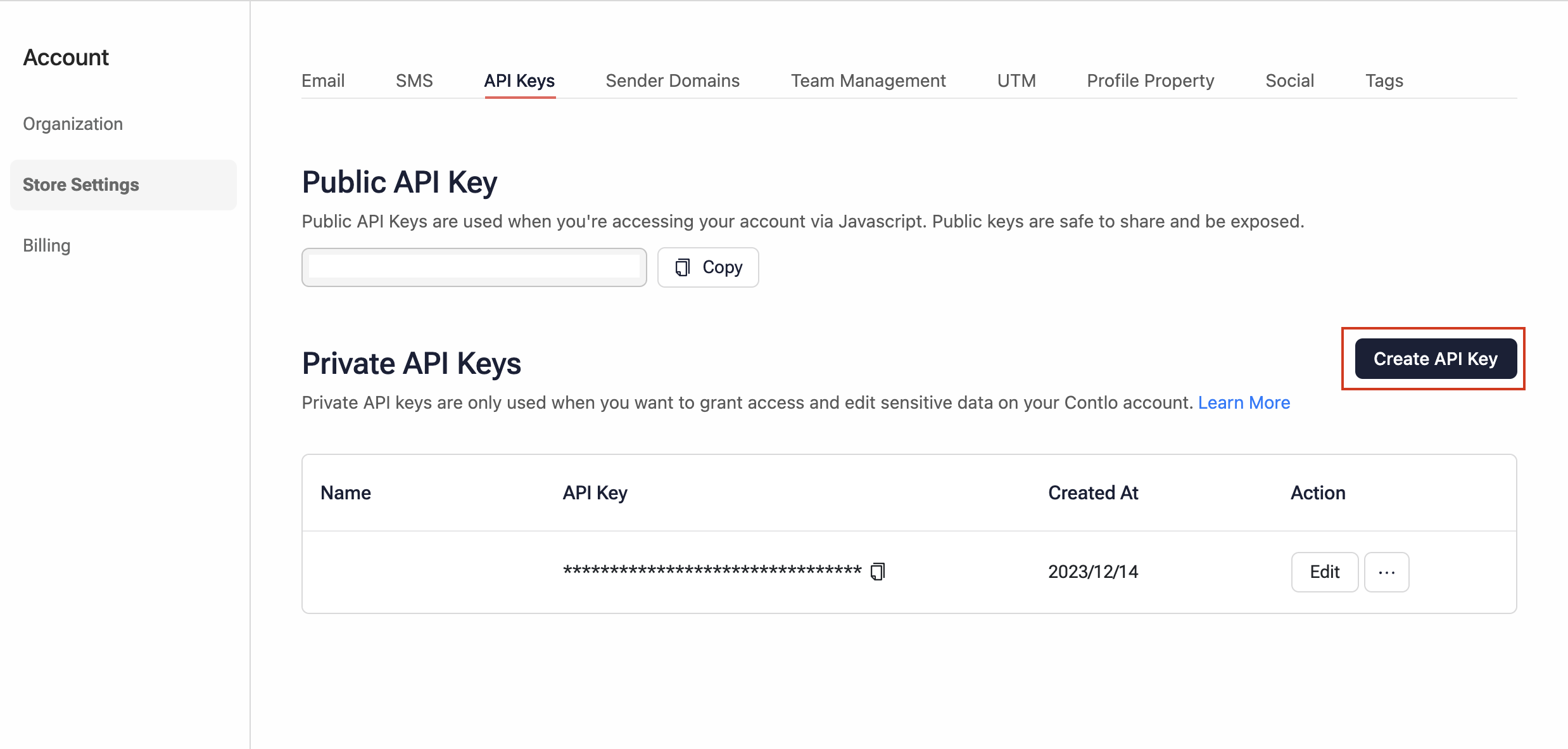
- Copy the newly created API key to add it later during the SDK integration.
Install Mobile push
- Go to App Market from the sidebar and search Mobile Push.
- Click Install.
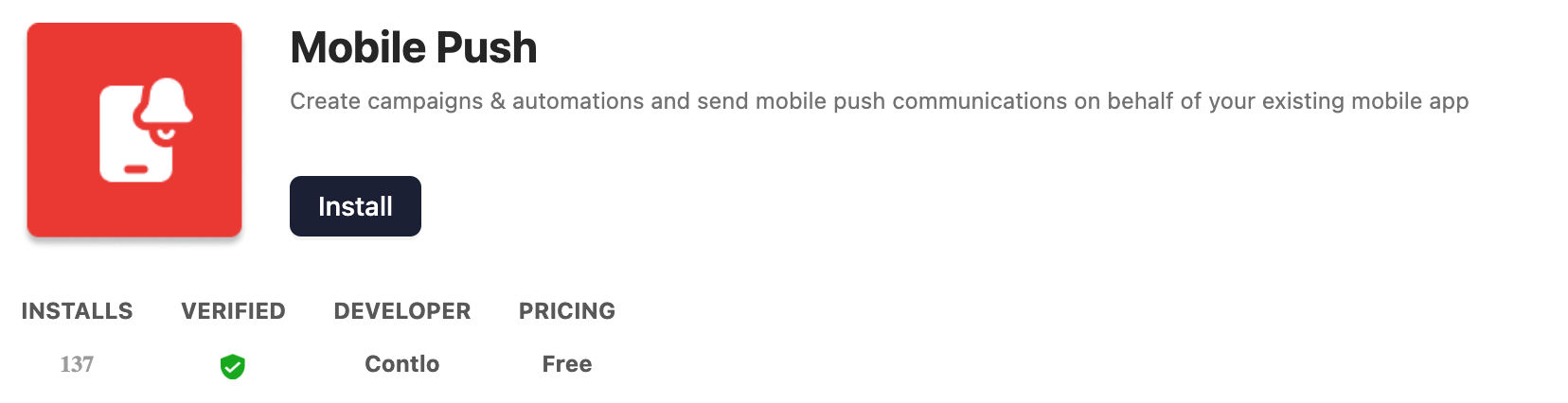
Installation
To install the Contlo SDK in your Android project, follow the steps below:
- If you are using Gradle 6.7 or lower, add the following code to your root build.gradle file:
allprojects {
repositories {
maven {
url 'https://nexus.contlo.com/repository/contlo-android-sdk'
}
}
}
- If you are using Gradle 6.8 or higher, add the following to your settings.gradle file:
dependencyResolutionManagement {
repositories {
maven {
url 'https://nexus.contlo.com/repository/contlo-android-sdk' }
}
}
- Add the following dependency to your module build.gradle file:
dependencies {
implementation 'com.contlo:android-core:1.0.0'
}
- Sync your build to install Contlo in your application.
Initialization
Once the installation is complete, follow the steps below to initialize the Contlo SDK:
- Open the BaseApplication file of your project that represents the start of the application and add the Contlo's
init()
method in the onCreate function of your Application class with following parameters:- Context: Pass the application context as
this
keyword. - API key: Pass the API key (created from the Contlo dashboard) as the parameter in the
init()
method.
- Context: Pass the application context as
- Add the
registerActivityLifecycleCallbacks
method to listen to the system events. Contlo SDK will take care of system events for you.
import android.app.Application
import com.contlo.androidsdk.main.Contlo
import com.contlo.androidsdk.lifecycle.ContloSDKLifecycleCallbacks
class ContloApplication : Application() {
override fun onCreate() {
super.onCreate()
Contlo.init(this, "<API_KEY>")
registerActivityLifecycleCallbacks(ContloSDKLifecycleCallbacks(applicationContext))
}
}
Verify Integration:
After initializing the SDK, open your application on a device or emulator.
Go to the Audiences section on your Contlo dashboard. You would see a new anonymous user is created.
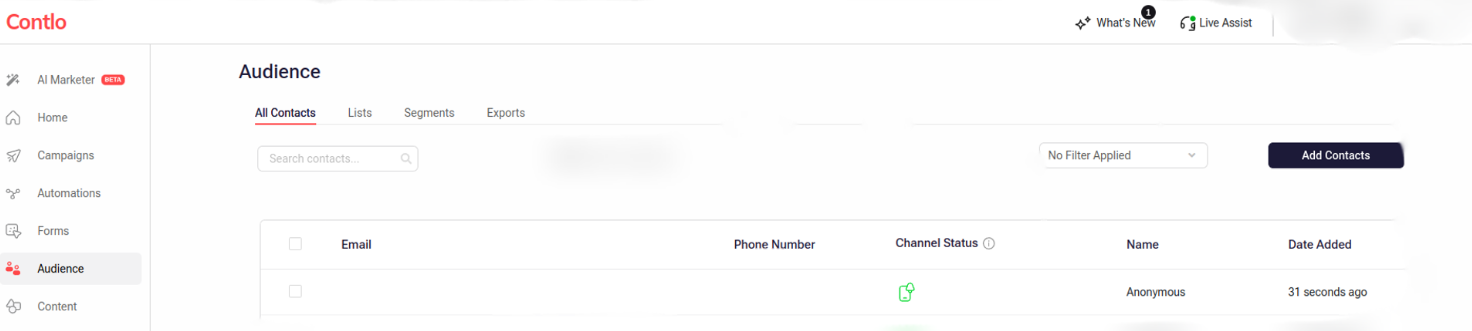
You have successfully integrated the Contlo Android SDK.
Welcome aboard!